Hibernate - Inheritance – Strategy 2 ( with Annotation ) : Table Per Sub Class Hierarchy
Hibernate
Annotation ( Inheritance – Strategy 2 : Table Per Sub Class
Hierarchy ) Application
Inheritance
Mapping
Hibernate
supports 3 basic inheritance mapping strategies.
1.
Table per class hierarchy
2.
Table per subclass
3.
Table per concrete class
For this example we use below assotations. @Inheritance( strategy = InheritanceType.JOINED) in the parent class and @PrimaryKeyJoinColumn( name = "empId" ) annotation in the sub class
For this example we use below assotations. @Inheritance( strategy = InheritanceType.JOINED) in the parent class and @PrimaryKeyJoinColumn( name = "empId" ) annotation in the sub class
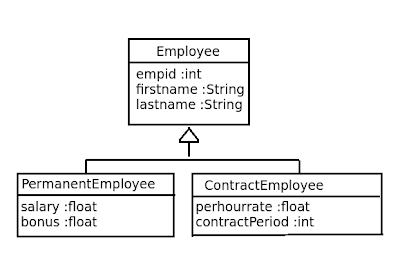
Suppose
you have the base Employee class, with PermanentEmployee
and ContractEmployee as subclasses.
Steps -
Steps -
Step
1 - Create POJO classes ( Employee.java and PermanentEmployee,
ContractEmployee sub-classes )
Step
2 - Hibernate Configurations ( hibernate.cfg.xml )
Step
3
- Create class to do the execution ( ExecuteEmployee.java )
Step 1 - Create POJO classes
Step 1 - Create POJO classes
Create POJO (Plain Old Java Object) classes that represents the Employee, PermanentEmployee and ContractEmployee.
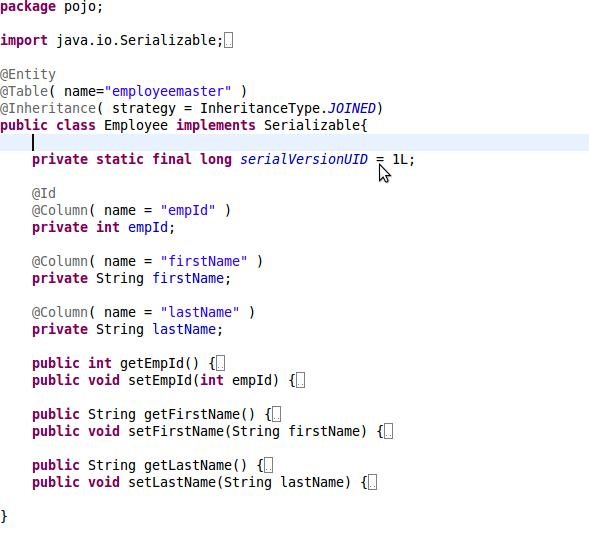
Step 2 - Hibernate Configurations
Create
configuration file name as hibernate.cfg.xml and save it.
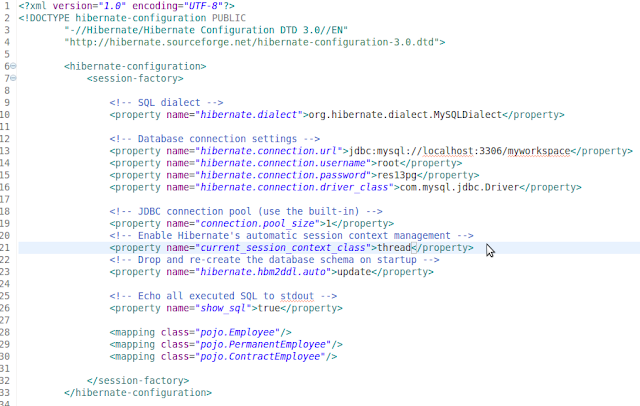
Step 3 - Create Execute class
Create a ExecuteEmployee class to do the execution.
package
impl;
import
java.util.Iterator;
import
java.util.List;
import
org.hibernate.Session;
import
org.hibernate.Transaction;
import
org.hibernate.cfg.AnnotationConfiguration;
import
pojo.ContractEmployee;
import
pojo.Employee;
import
pojo.PermanentEmployee;
public
class
ExecuteEmployee {
/**
* @param
args
*/
public
static
void
main(String[] args) {
ExecuteEmployee
executeEmployee = new
ExecuteEmployee();
executeEmployee.addEmployee();
executeEmployee.listEmployee();
}
private
void
addEmployee(){
Transaction
transaction = null;
Session
hbSession = new
AnnotationConfiguration().configure("config/hibernate.cfg.xml")
.buildSessionFactory().openSession();
try{
transaction
= hbSession.beginTransaction();
Employee
employee = new
Employee();
employee.setEmpId(100100);
employee.setFirstName("Sanjeeva");
employee.setLastName("Pathirana");
PermanentEmployee
permanentEmployee = new
PermanentEmployee();
permanentEmployee.setEmpId(100101);
permanentEmployee.setFirstName("Sandamali");
permanentEmployee.setLastName("Silva");
permanentEmployee.setSalary(50000);
permanentEmployee.setBonus(2500);
ContractEmployee
contractEmployee = new
ContractEmployee();
contractEmployee.setEmpId(100102);
contractEmployee.setFirstName("Thinuli");
contractEmployee.setLastName("Pathirana");
contractEmployee.setPerhourrate(150);
contractEmployee.setContractperiod(6);
hbSession.save(employee);
hbSession.save(permanentEmployee);
hbSession.save(contractEmployee);
hbSession.getTransaction().commit();
System.out.println("Insert
All Employees ");
}catch
(Exception e) {
if(null
!= transaction){
transaction.rollback();
}
e.printStackTrace();
}finally{
hbSession.flush();
hbSession.close();
}
}
private
void
listEmployee(){
Transaction
transaction = null;
Session
hbSession = new
AnnotationConfiguration().configure("config/hibernate.cfg.xml")
.buildSessionFactory().openSession();
try{
transaction
= hbSession.beginTransaction();
@SuppressWarnings("unchecked")
List<Employee>
employeesList = hbSession.createQuery("FROM
Employee").list();
for(Iterator<Employee>
iterator = employeesList.iterator(); iterator.hasNext();){
Employee
employee = iterator.next();
System.out.println("");
System.out.println("EmpId :
" + employee.getEmpId());
System.out.println("FirstName :
" + employee.getFirstName());
System.out.println("LastName :
" + employee.getLastName());
if(employee
instanceof
PermanentEmployee ){
System.out.println("Salary :
" +
((PermanentEmployee)employee).getSalary() );
System.out.println("Bonus :
" +
((PermanentEmployee)employee).getBonus() );
}else{
System.out.println("Salary :
- " );
System.out.println("Bonus :
- ");
}
if(employee
instanceof
ContractEmployee ){
System.out.println("Perhourrate :
" +
((ContractEmployee)employee).getPerhourrate());
System.out.println("Contract
Period : " +
((ContractEmployee)employee).getContractperiod() );
}else{
System.out.println("Perhourrate :
- ");
System.out.println("Contract
Period : - ");
}
}
hbSession.getTransaction().commit();
}catch
(Exception e) {
if(null
!= transaction){
transaction.rollback();
}
e.printStackTrace();
}finally{
hbSession.flush();
hbSession.close();
}
}
}
Out
put on the above application as follows.
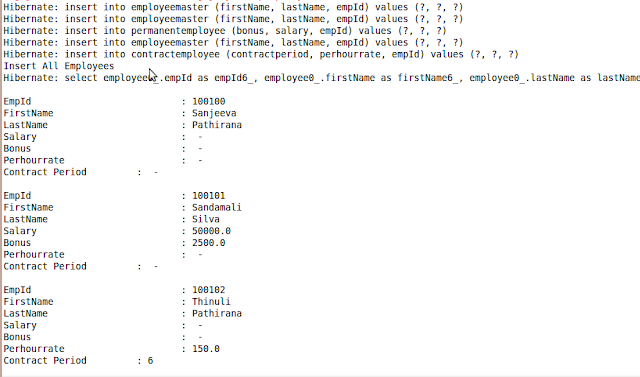